How To Use Strapi With React
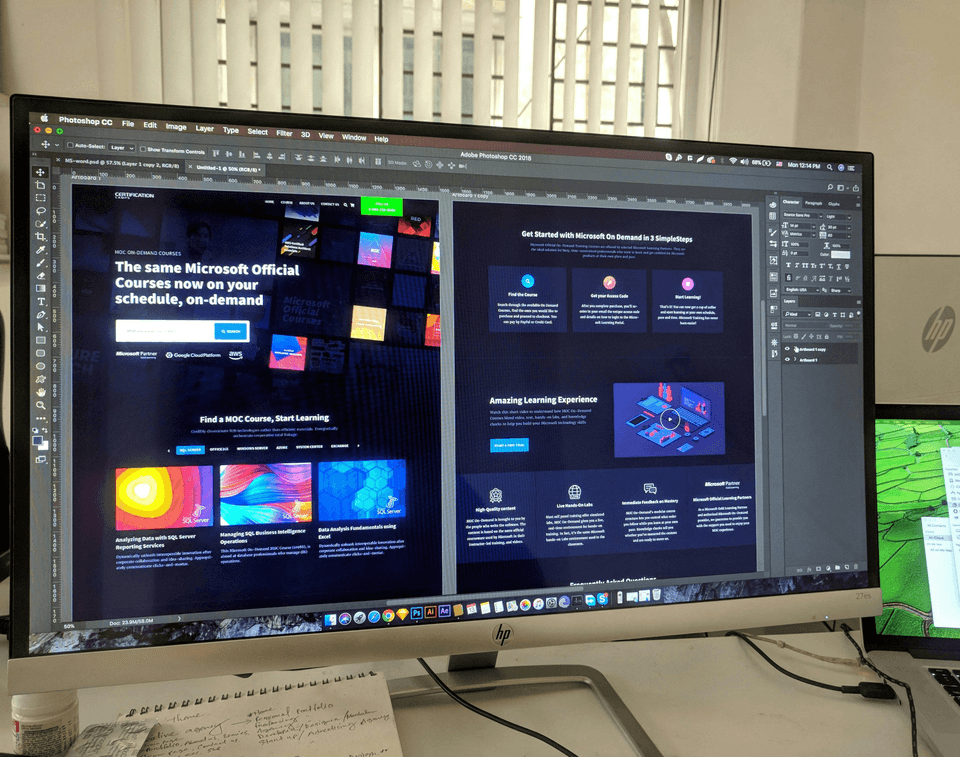
Strapi is a powerful open-source headless CMS (Content Management System) that allows developers to easily create, manage, and deliver content across various platforms. When combined with React, a popular JavaScript library for building user interfaces, Strapi becomes even more versatile and efficient. In this article, we will explore how to use Strapi with React to create dynamic and interactive web applications. Getting Started with Strapi and React To begin using Strapi with React, you will need to set up a Strapi backend and a React frontend. The first step is to install Strapi by running the following command in your terminal: ` npx create-strapi-app my-project --quickstart ` This will create a new Strapi project with a quickstart template. Next, you will need to start the Strapi server by running: ` cd my-project npm run develop ` Once the Strapi server is up and running, you can start creating content types and adding content to your Strapi backend. This can be done through the Strapi admin panel, which is accessible at http://localhost:1337/admin. Now that you have set up your Strapi backend, it's time to create a React frontend to interact with the Strapi API. You can start by creating a new React project using Create React App: ` npx create-react-app my-app cd my-app ` Next, you will need to install Axios, a popular library for making HTTP requests, to handle API calls to your Strapi backend: ` npm install axios ` Now you can start building your React components and fetching data from your Strapi backend. Here's an example of how you can fetch and display data from your Strapi API in a React component: `jsx import React, { useState, useEffect } from 'react'; import axios from 'axios'; const MyComponent = () => { const [data, setData] = useState([]); useEffect(() => { const fetchData = async () => { const response = await axios.get('http://localhost:1337/posts'); setData(response.data); }; fetchData(); }, []); return (
{post.title}
{post.content}